Published
- 4 min read
C-Chalk for pretty terminals
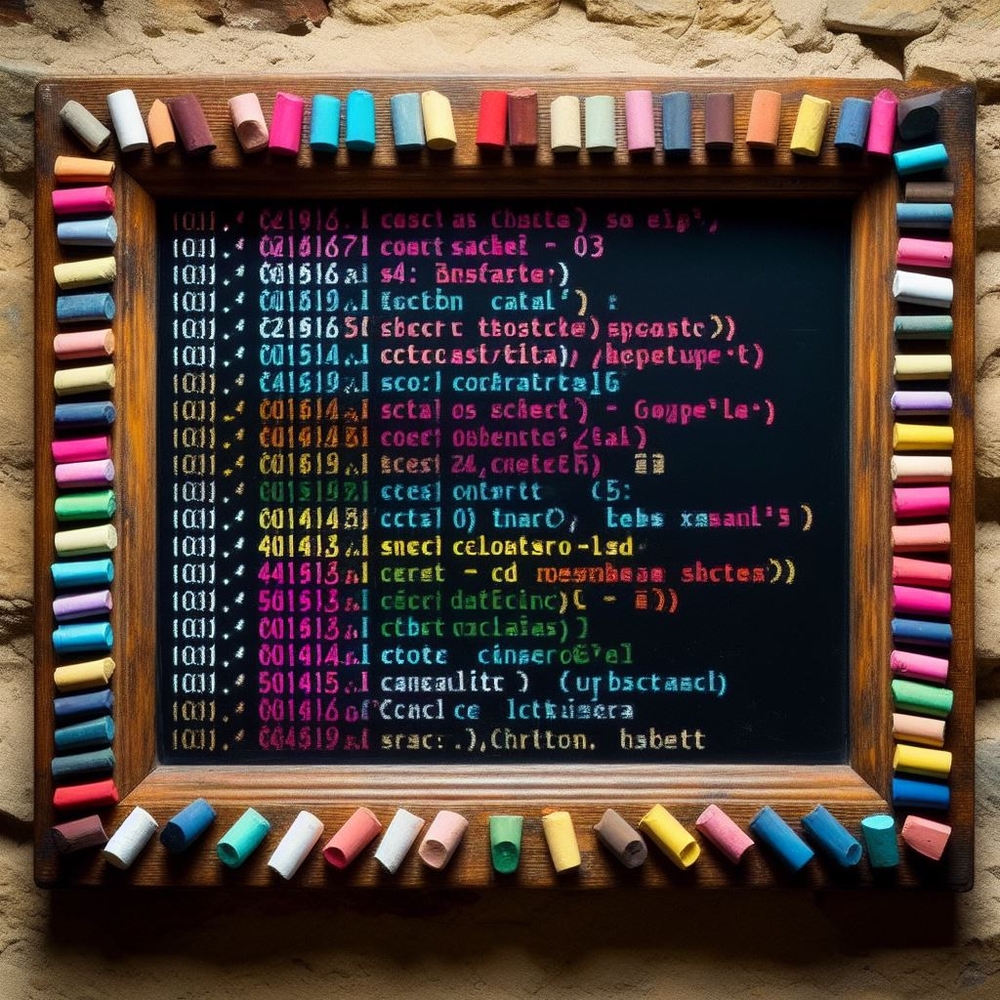
C-Chalk
C-Chalk is a C library that allows you to print terminal outputs in multiple colors. I use this library when I do embedded development on the ESP32, but it can be used with any C / C++ application. The repo can be located at https://github.com/Mair/c-chalk
Motivation
The ESP32 interacts very well with the console and outputs standard printf statements. In addition, the ESP32 comes with a nice logging library that can print informational messages in green, warnings in yellow and errors in red (see this introduction for more details).
In complex applications where there are multiple tasks going on at the same time, its nice to have an eye catching color that indicates important output.
Now with C-Chalk
Another interesting use case would be for creating interactive terminals.
Installation
All you need to have to get C-Chalk to work is the c and h files located in the components directory or you can clone the repo with git clone https://github.com/Mair/c-chalk.git
and add the components directory to your project. If you already have a components directory, add the components/cchalk folder to your components directory
Usage
Use With Named Colors
In the cchalk.h file there is an enumerator with predefined colors prefixed with CHALK_. To print something in yellow, you can use
chalk_printf(CHALK_YELLOW, "some yellow text\n");
New Line Insertion
Most functions have a _ln optional suffix to automatically print a new line.
chalk_printf_ln(CHALK_RED, "some red text with a new line added");
Note that if you use a function with an _ln suffix you can omit
"\n"
at the end of the line.
The library functions use the same functionality as printf
chalk_printf_ln(CHALK_GREEN, "this is green and is line number %d", 3);
You can add as many parameters as you like:
Background Color
You can use chalk_printf_background_ln to print a foreground and a background color:
chalk_printf_background_ln(CHALK_BLACK, CHALK_WHITE, "this is black text on a white background");
NB: When printing the background, it is recommended to use the _ln version of the function, otherwise you might get color bleeding into the next line
chalk_printf_background(CHALK_BLACK, CHALK_WHITE, "this will bleed color into the next line\n");
This is because the end of the color is only applied after the new line.
Use with RGB Colors
For printing colors with RGB the following functions are available:
void chalk_rgb(uint8_t r, uint8_t g, uint8_t b, uint8_t bg_r, uint8_t bg_g, uint8_t bg_b, const char *format, ...);
void chalk_rgb_ln(uint8_t r, uint8_t g, uint8_t b, uint8_t bg_r, uint8_t bg_g, uint8_t bg_b, const char *format, ...);
void chalk_printf_foreground_rgb(uint8_t r, uint8_t g, uint8_t b, const char *format, ...);
void chalk_printf_foreground_rgb_ln(uint8_t r, uint8_t g, uint8_t b, const char *format, ...);
Here is an example:
chalk_printf_foreground_rgb(0, 0, 125, "this is text using rgb values\n");
chalk_rgb_ln(255, 100, 100, 50, 255, 50, "this is text and background using rgb values");
Text Decorations
Text decorations don’t work on all terminals so its a good idea to test it.
Here is an example of using text decorations:
chalk_printf_ln(CHALK_BOLD, "text with CHALK_BOLD decoration");
chalk_printf_ln(CHALK_UNDERLINE, "text with CHALK_UNDERLINE decoration");
chalk_printf_ln(CHALK_DIM, "text with CHALK_DIM decoration");
chalk_printf_ln(CHALK_REVERSE, "text with CHALK_REVERSE decoration");
chalk_printf_ln(CHALK_STRIKETHROUGH, "text with CHALK_STRIKETHROUGH decoration");
Altogether now
#include <stdio.h>
#include "cchalk.h"
// #include "esp_log.h"
void app_main(void)
{
// ESP_LOGI("main", "A log message");
// ESP_LOGI("main", "Another message I don't core about");
// ESP_LOGI("main", "And yet another");
// chalk_printf_background_ln(CHALK_DARKBLUE, CHALK_CYAN, "finally something I do care about");
// ESP_LOGI("main", "Some other message I don't care about");
chalk_printf(CHALK_YELLOW, "some yellow text\n");
chalk_printf_ln(CHALK_RED, "some red text with a new line added");
chalk_printf_ln(CHALK_GREEN, "this is green and is line number %d", 3);
chalk_printf_background_ln(CHALK_BLACK, CHALK_WHITE, "this is black text on a white background");
// chalk_printf_background(CHALK_BLACK, CHALK_WHITE, "this will bleed color into the next line\n");
chalk_printf_foreground_rgb(0, 255, 255, "this is text using rgb values\n");
chalk_rgb_ln(255, 100, 100, 50, 255, 50, "this is text and background using rgb values");
chalk_printf_ln(CHALK_BOLD, "text with CHALK_BOLD decoration");
chalk_printf_ln(CHALK_UNDERLINE, "text with CHALK_UNDERLINE decoration");
chalk_printf_ln(CHALK_DIM, "text with CHALK_DIM decoration");
chalk_printf_ln(CHALK_REVERSE, "text with CHALK_REVERSE decoration");
chalk_printf_ln(CHALK_STRIKETHROUGH, "text with CHALK_STRIKETHROUGH decoration");
}
pull requests for the repo are welcome